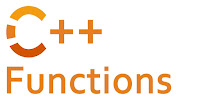
PLUS ONE COMPUTER SCIENCE
First Year Computer Science Previous Questions Chapter wise ..
Chapter 10. Functions
1. Which one of the following is NOT equal to others?
a) pow(64,...
For Plus 1 & Plus 2 Computer Students ( Content is progressing ..Keep visiting)
Previous Question paper answered , and Questions from Textbook
Previous Question paper answered , and Questions from Textbook
Previous Question paper answered , and Questions from Textbook.
Previous Question paper answered , and Questions from Textbook
Contact : +91 9036433020 , mail2trueiq@gmail.com