PLUS ONE COMPUTER SCIENCE
First Year Computer Science Previous Questions Chapter wise ..
Chapter 10. Functions
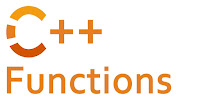
1. Which one of the following is NOT equal to others?
a) pow(64, 0.5)
b) pow(2,3)
c) sqrt(64)
d) pow(3,2)
Ans. a
2. Write a recursive C++ function that returns sum of the first n natural numbers.
#include<iostream>
using namespace std;
int add(int n);
int main()
{
int n;
cout << "Enter a positive integer: ";
cin >> n;
cout << "Sum = " << add(n);
return 0;
}
int add(int n)
{
if(n != 0)
return n + add(n - 1);
return 0;
}
Output
Enter an positive integer: 10 Sum = 55
3. List any three string functions in C++ and specify the value returned by them.
strlen( )
strlen(string) To find the length of a string.
strcpy( )
strcpy(string1, string2) To copy one string into another
strcmp( )
strcmp(string1, string2) To compare two strings.
Returns 0 if string1 and string2 are same.
Returns a –ve value if string1 is alphabetically lower than string2.
Returns a +ve value if string1 is alphabetically higher than string2.
4. Name the built in function to check whether a character is alphanumeric or not.
Ans. isalpha( )
5. Read the function definition given below. Predict the output, if the function is called as
convert(7);
void convert(int n)
{
if (n>1)
convert(n/2);
cout<<n%2;
}
6. Explain the difference between call-by-value method and call-by-reference method with
the help of examples.
Call by value
Call by value method copies the value of an argument into the formal parameter of that function. Therefore, changes made to the parameter of the main function do not affect the argument.
In this parameter passing method, values of actual parameters are copied to function's formal parameters, and the parameters are stored in different memory locations. So any changes made inside functions are not reflected in actual parameters of the caller.
void change(int n)
{
n = n + 1;
cout << "n = " << n << '\n';
}
int main()
{
int x = 20;
change(x);
cout << "x = " << x;
}
The following will be the output of the above code:
n = 21
x = 20
Call by reference
Call by reference method copies the address of an argument into the formal parameter. In this method, the address is used to access the actual argument used in the function call. It means that changes made in the parameter alter the passing argument.
In this method, the memory allocation is the same as the actual parameters. All the operation in the function are performed on the value stored at the address of the actual parameter, and the modified value will be stored at the same address.
void change(int & n)
{
n = n + 1;
cout << "n = " << n << '\n';
}
int main()
{
int x=20;
change(x);
cout << "x = " << x;
}
Output
n = 21
x = 21
7. Arguments used in call statement are formal arguments. State true or false.
Ans, True.
Arguments or parameters are the means to pass values from the calling function to the called function. The variables used in the function definition as arguments are known as formal arguments.
8. Differentiate the string functions strcmp() and strcmpi().
The strcmpi() function is same as that of the strcmp() function but the only difference is that strcmpi() function is not case sensitive and on the other hand strcmp() function is the case sensitive
strcmp(string1, string2) To compare two strings.
Returns 0 if string1 and string2 are same.
Returns a –ve value if string1 is alphabetically lower than string2.
Returns a +ve value if string1 is alphabetically higher than string2.
strcmpi( string1,string2) To compare two strings.If same Returns 0 otherwise 1.
9. Differentiate break and continue statements in C++.
10. Explain recursive function with the help of a suitable example.
Recursion is the process of calling a function by itself and the function is known as recursive function.
12. Suggest most suitable built-in function in C++ to perform the following tasks:
(a) To find the answer for 5^3
.
(b) To find the number of characters in the string “KERALA”.
(c) To get back the number 10 if the argument is 100.
Ans. a) pow(5,3);
b) n= strlen("KERALA");
c) sqrt(100);
13. A function can call itself for many times and return a result.
(a) What is the name given to such a function?
(b) Write a function definition of the above type to find the sum of natural numbers
from 1 to N. (Hint: If the value of N is 5, the answer will be 1+2+3+4+5=15)
Ans. a) Recursive function
Ref Qn no.2
14. Explain two types of variable according to its scope and life.
Local variable
• Declared within a function or a block of statements.
• Available only within that function or block.
• Memory is allocated when the function or block is active and freed when the execution of the function or block is completed.
Global variable
• Declared within a function or a block of statements and defined after the calling function.
• Accessible only within that function or the block.
• Memory is allocated just before the execution of the program and freed when the program stops execution.
15. Write a C++ Program to display the simple interest using function.
#include<iostream>
16. The function which calls itself is called a _______.
Ans. Recursion
17. Construct the function prototype for the following functions:
(a) The function Display() accepts one argument of type double and does not return any
value.
(b) Total() accepts two arguments of type int, float respectively and return a float type
value.
18. Name the different methods used for passing arguments to a function. Write the
difference between them with examples.
Ref.Qn no.6
7. Arguments used in call statement are formal arguments. State true or false.
Ans, True.
Arguments or parameters are the means to pass values from the calling function to the called function. The variables used in the function definition as arguments are known as formal arguments.
8. Differentiate the string functions strcmp() and strcmpi().
The strcmpi() function is same as that of the strcmp() function but the only difference is that strcmpi() function is not case sensitive and on the other hand strcmp() function is the case sensitive
strcmp(string1, string2) To compare two strings.
Returns 0 if string1 and string2 are same.
Returns a –ve value if string1 is alphabetically lower than string2.
Returns a +ve value if string1 is alphabetically higher than string2.
strcmpi( string1,string2) To compare two strings.If same Returns 0 otherwise 1.
9. Differentiate break and continue statements in C++.
Both “break” and “continue” are the ‘jump’ statements, that transfer control of the program to another part of the program. The main difference between break and continue is that break is used for immediate termination of loop. On the other hand, ‘continue’ terminate the current iteration and resumes the control to the next iteration of the loop.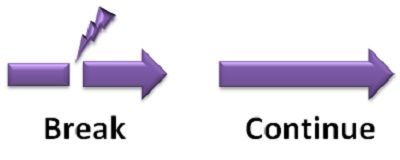
The break statement is primarily used as the exit statement, which helps in escaping from the current block or loop. Conversely, the continue statement helps in jumping from the current loop iteration to the next loop. C++ supports four jump statements, namely ‘return’, ‘goto’, ‘break’ and ‘continue’.
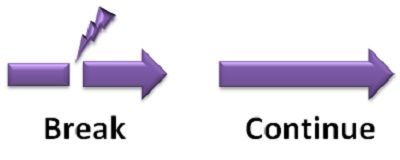
The break statement is primarily used as the exit statement, which helps in escaping from the current block or loop. Conversely, the continue statement helps in jumping from the current loop iteration to the next loop. C++ supports four jump statements, namely ‘return’, ‘goto’, ‘break’ and ‘continue’.
10. Explain recursive function with the help of a suitable example.
Recursion is the process of calling a function by itself and the function is known as recursive function.
12. Suggest most suitable built-in function in C++ to perform the following tasks:
(a) To find the answer for 5^3
.
(b) To find the number of characters in the string “KERALA”.
(c) To get back the number 10 if the argument is 100.
Ans. a) pow(5,3);
b) n= strlen("KERALA");
c) sqrt(100);
13. A function can call itself for many times and return a result.
(a) What is the name given to such a function?
(b) Write a function definition of the above type to find the sum of natural numbers
from 1 to N. (Hint: If the value of N is 5, the answer will be 1+2+3+4+5=15)
Ans. a) Recursive function
Ref Qn no.2
14. Explain two types of variable according to its scope and life.
Local variable
• Declared within a function or a block of statements.
• Available only within that function or block.
• Memory is allocated when the function or block is active and freed when the execution of the function or block is completed.
Global variable
• Declared within a function or a block of statements and defined after the calling function.
• Accessible only within that function or the block.
• Memory is allocated just before the execution of the program and freed when the program stops execution.
15. Write a C++ Program to display the simple interest using function.
#include<iostream>
using namespace std;
class Simple_interest {
public:
float si, amount, r;
void calculate(float amt, float rate) {
amount = amt;
r = rate;
}
void calculate(float time) {
si = (amount * r * time) / 100;
cout << "\nSimple interest is : " << si;
}
};
int main() {
float amt, rate, time;
cout << "Enter amount : ";
cin>>amt;
cout << "\nEnter rate : ";
cin>>rate;
cout << "\nEnter time : ";
cin>>time;
Simple_interest obj;
obj.calculate(amt, rate);
obj.calculate(time);
return 0;
}
Output:
Enter amount : 200
Enter rate : 5
Enter time : 3
Simple interest is : 30
Ans. Recursion
17. Construct the function prototype for the following functions:
(a) The function Display() accepts one argument of type double and does not return any
value.
(b) Total() accepts two arguments of type int, float respectively and return a float type
value.
18. Name the different methods used for passing arguments to a function. Write the
difference between them with examples.
Ref.Qn no.6