PLUS TWO COMPUTER APPLICATION
Second Year Computer Application(Commerce) Previous Questions Chapter wise ..
Chapter 2: Arrays
1. char s1[10] =”hello”, s2[];
strcpy(s1,s2);
cout<<s2;
What will be the output?
- a) hello
- b) hel
- c)hell
- d) no output
2. How memory is allocated for a float array?
If it is a float array then its elements will occupy 8 bytes of memory each. But this is not the total size or memory allocated for the array. They are the sizes of individual elements in the array.
3. How we can initialize an integer array ?Give an example?
Giving values to the array elements at the time of array declaration is known as array initialization .
Example An array of 10 scores: 89, 75, 82, 93, 78, 95, 81, 88, 77,and 82
int score[10] = {89, 75, 82, 93, 78, 95, 81, 88, 77, 82};
4. Define an array.Give an example of an integer array declaration?
Arrays are used to store a set of values of the same type in contiguous memory locations under a single variable name. Each element in an array can be accessed using its position in the list, called index number or subscript.
Score of 100 marks : int score[100];
5. Write C++ initialization statement to initialize an integer array name ‘MARK’ with the values 70, 80 , 85 , 90.
int MARK[4]={70,80,85,90};
6 . Explain how allocation of string takes place in memory?
A sting can be considered as an array of characters.Character array ,characters will occupy 1 byte of memory each.Null character('/0') is stored in the end of the string.
example: "computer" is a string.It can be stored in memory by 1byte of each character.
7. Explain gets( ) and puts( ) functions?
gets() function is used to accept a string of characters including whitespace from standard input device(eg. Keyboard) and store it in a character array.
Syntax: gets(String_data)
Eg. gets(str);
puts() function is used to display a string data on a standard output device(eg. Monitor)
Syntax: puts(String_data)
Eg. puts(“ Hello “);
8 . Consider the following C++ code
char text[20];
cin≫text; cout≪text;
If the input string is “Computer Programming”; what will be the output ?justify your answer.
Ans. output- Computer Programming
Character array name text is the size 20.That is 20 characters can allocate to the memory.If the input string is "computer programming"total characters are 20 including one white space between computer and programming.so the output will print computer programming.Null character is stored at the end of the string.
9. Write the output of the C++ code segment
char s1[10] = “Computer”;
char s2[10] = “Application”;
strcpy(s1,s2);
cout<< s2;
Output : -Applicatio
10. Write a C++ program to accept a string and find its length without using built in function. Use a character array to store the string .
/* C Program to find the length of a String without using built in function
* using any standard library function */ #include <stdio.h> int main() { /* Here we are taking a char array of size * 100 which means this array can hold a string * of 100 chars. You can change this as per requirement */ char str[100],i; printf("Enter a string: \n"); scanf("%s",str); // '\0' represents end of String for(i=0; str[i]!='\0'; ++i); printf("\nLength of input string: %d",i); return 0; }
output
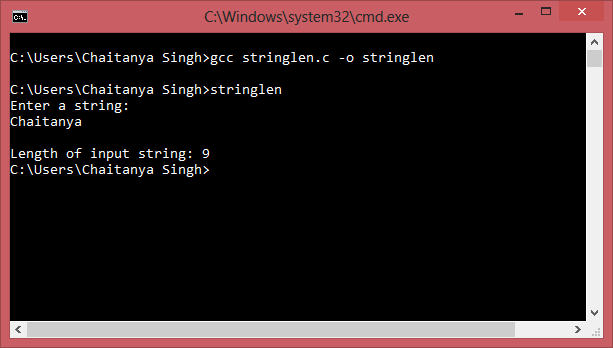
11. Write a program to input ‘N’ numbers into an integer array and find the largest number in the array.
#include <iostream>
using namespace std;
- int main()
{
int i, n;
float arr[100];
- cout << "Enter total number of elements(1 to 100): ";
cin >> n;
cout << endl;
- // Store number entered by the user
for(i = 0; i < n; ++i)
{
cout << "Enter Number " << i + 1 << " : ";
cin >> arr[i];
}
- // Loop to store largest number to arr[0]
for(i = 1;i < n; ++i)
{
// Change < to > if you want to find the smallest element
if(arr[0] < arr[i])
arr[0] = arr[i];
}
cout << "Largest element = " << arr[0];
- return 0;
}
output
Enter total number of elements: 8
Enter Number 1: 23.4 Enter Number 2: -34.5 Enter Number 3: 50 Enter Number 4: 33.5 Enter Number 5: 55.5 Enter Number 6: 43.7 Enter Number 7: 5.7 Enter Number 8: -66.5 Largest element = 55.512. Write C++ program to input 10 numbers in an integer array and find the sum of numbers which are exact multiples of 5.
13. Write C++ program to input 10 numbers in an integer array and find the second largest element.
#include <iostream> using namespace std; int main(){ int n, num[50], largest, second; cout<<"Enter number of elements: "; cin>>n; for(int i=0; i<n; i++){ cout<<"Enter Array Element"<<(i+1)<<": "; cin>>num[i]; } /* Here we are comparing first two elements of the * array, and storing the largest one in the variable * "largest" and the other one to "second" variable. */ if(num[0]<num[1]){ largest = num[1]; second = num[0]; } else{ largest = num[0]; second = num[1]; } for (int i = 2; i< n ; i ++) { /* If the current array element is greater than largest * then the largest is copied to "second" and the element * is copied to the "largest" variable. */ if (num[i] > largest) { second = largest; largest = num[i]; } /* If current array element is less than largest but greater * then second largest ("second" variable) then copy the * element to "second" */ else if (num[i] > second && num[i] != largest) { second = num[i]; } } cout<<"Second Largest Element in array is: "<<second; return 0; }
Output:
Enter number of elements: 5 Enter Array Element1: 12 Enter Array Element2: 31 Enter Array Element3: 9 Enter Array Element4: 21 Enter Array Element5: 3 Second Largest Element in array is: 21
Explanation:
User enters 5 as the value of n, which means the first for loop ran times to store the each element entered by user to the array, first element in num[0], second in num[1] and so on.
14. Consider the following C++ code
int main()
{
char str[20];
cout<<"Enter the string";
cin>>str;
puts(str);
return 0;
}
a) Write the value of str if the string "HELLO WORLD" is input to the code.Justify?
Ans. HELLO
The letters of the word HELLO is stores in the array str as first 6 char elements The input is completed due to the blank space and the word "WORLD" is skipped
b) Write the amount of memory allocated for storing the arr str.Give reason?
The amount of memory allocated for storing the arr str is 20.
Note that a null character '\0' is stored at the end of the string. This character is used as the string terminator and added at the end automatically. Thus we can say that memory required to store a string will be equal to the number of characters in the string plus one byte for null character.
c) Write an alternative we can use to input the string in place of cout?
Ans. gets(str );
15. Write a C++ statement to declare with an array size 25 to accept the name of a student
Ans. char name[25];
16. -------------- is a collection of elements of same type place in a contigious memory locations
Ans. Array
17. Write C++ statement for the following
a) Declare an integers array "MARK" to store marks of five pupils
b) Initialize the array "MARK" with values 30,50,65,25,70
Ans.a) int MARK[5];
b) int MARK[5]={30,50,65,25,70};
15. Write a C++ statement to declare with an array size 25 to accept the name of a student
Ans. char name[25];
16. -------------- is a collection of elements of same type place in a contigious memory locations
Ans. Array
17. Write C++ statement for the following
a) Declare an integers array "MARK" to store marks of five pupils
b) Initialize the array "MARK" with values 30,50,65,25,70
Ans.a) int MARK[5];
b) int MARK[5]={30,50,65,25,70};