PLUS ONE
COMPUTER APPLICATION / COMPUTER SCIENCE
Chapter 5 (6 for CS) Data Types And Operators
(+1. Computer Application Questions and answers from text book)
PLUS ONE
COMPUTER APPLICATION / COMPUTER SCIENCE 1. Predict the output of the following operations if x=5 and y=3.
a. x>=10 && y>=4
b. x>=1 && y>=3
c. x>5 && y>=2
d. x<10 && y>2
e. x<10 && y>x
f. x>=10 || y>=4
g. x>=1 || y>=3
h. x>5 || y>=2
i. x<10 || y>2
j. x<10 || y>x
Ans. a. FALSE
b. TRUE
c. FALSE
d. TRUE
e. FALSE
f. FALSE
g. TRUE
h. TRUE
i. TRUE
j. TRUE
2. Predict the output if p=5, q=3, r=2
a. p-q*r/2
b. p*q/r
c. p-q-r*2+p
d. p+5*q+r*r/2
Ans. a. 5-3*2/2 = 5-3*1 =2
b. 5*3/2 =7.5
c. 5-3-2*2+5 =5-3-4+5 =10-7=3
d. 5+5*3+2*2/2 = 5+15+2 =22
3. What are data types? List all predefined data types in C++?
Data types are the means to identify the nature of the data and the set of operations that can be performed on the data.
There are 5 types of Fundamental data types,
1). Char: They are symbols covered by the character set of C++ language. It uses only one byte of memory (storing in memory with its ASCII value).
2). Int : Whole numbers without fractional part. It can be positive or negative, or zero. It uses Four bytes of memory based on GCC compiler.
3). Float : They are numbers with fractional part. It uses four bytes of memory. It can be represented either by scientific notation or mantissa exponential method.
4). Double: It is used for handling large floating point numbers. It uses 8 bytes of memory.
5). Void : uses zero bytes of memory.
4. What is the use of void data type?
The data type void is a keyword and it indicates an empty set of data. Obviously it does not require any memory space.
5. What is a constant?
Constants are like a variable, except that their value never changes during execution once defined. ...Constants in C are the fixed values that are used in a program, and its value remains the same during the entire execution of the program. Constants are also called literals. Constants can be any of the data types.
6. What is dynamic initialization of variables?
Dynamic initialization is that in which initialization value isn't known at compile-time. It's computed at runtime to initialize the variable. Example, ... That is, static-initialization usually involves constant-expression (which is known at compile-time), while dynamic-initialization involves non-constant expression.
7. Define operators.
Tokens constituted by predefined symbols that can trigger to carry out operation. The participants of the operation are called operands. The operators either be constant or literal
6. What is meant by a unary operation?
only one operand( eg +(positive),-(negative)
example:+x,-y etc.
7. What is declaration statement?
It is used to declare the type of the variable or constant used in the program.
Eg. int x, y ; int a=10;
8. What is the input operator ">>" and output operator "<<" called ?
extract operator(>>) and insertion operator(<<)
9. What will be the result of a = 5/3 if a is
(i) float
(ii) int
Ans. i. 1.66
ii. 1
10. Write an expression that uses a relational operator to return true if the variable
total is greater than or equal to final.
11. Given that i =4, j =5, k =2 what will be the result of following expressions?
(i) j <k ------------ False
(ii) j <j ----------- False
(iii) j <= k ----------- False
(iv) i == j ------------ False
(v) i == k ----------- False
(vi) j > k ---------- True
(vii) j >= i ---------- True
(viii) j! = i ------------ True
(ix) j! = k --------------- True
(x) j <= k --------------- True
12. What will be the order of evaluation for following expressions?
(i) i+5>=j-6
(ii) s+10<p-2+2*q
Ans. i.
13. What will be the result of the following if ans is 6 initially?
(i) cout <<ans = 8 ;
(ii) cout << ans == 8
Ans. i.
14. What is a variable? List the two values associated with a variable.
Name given to a memory location is known as variable. It is use to store and retrieve data. It have three part: a) variable name b). Memory address(starting address of the allocated memory) c). Content 4
15. Explain logical operators.
and (&&): ( Result will be true only if both the combined expressions have true value).
or(||): Result will be true only if any of the combined expressions have true value ).
not(!): To get the negation of the give expression.
16. Find out the errors, if any, in the following C++ statements
(i) cout<< "a=" a;
(ii) m=5, n=l2;015
(iii) cout << "x" ; <
18. Explain the operators used in C++ in detail.
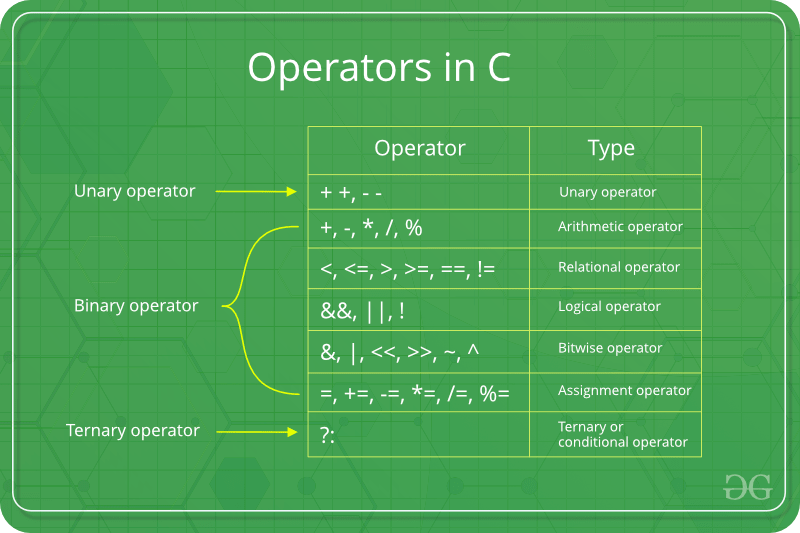
Operators are tokens constituted by predefined symbols that can trigger computer to carry out operations. The participants of an operation are called operands. An operand may be either a constant or a variable.For example, a+b triggers an arithmetic operation in which + (addition) is the operator and a, b are operands. Operators in C++ are classified based on various criteria. Based on number of operands required for the operation, operators are classified into three. They are unary, binary and ternary.
Unary operators : A unary operator operates on a single operand. Commonly used unary operators are unary+ (positive) and unary- (negative). These are used to represent the signs of a number. If we apply unary+ operator on a signed number, the existing sign will not change. If we apply unary- operator on a signed number, the sign of the existing number will be negated.
Binary operators: Binary operators operate on two operands. Arithmetic operators, relational operators, logical operators, etc. are the commonly used binary operators.
Ternary operator :Ternary operator operates on three operands. The typical example is the conditional operator (?:).
Arithmetic operators
Arithmetic operators are defined to perform basic arithmetic operations such as addition, subtraction, multiplication and division. The symbols used for this are +, -, * and / respectively. C++ also provides a special operator % (modulus operator) for getting remainder during division. All these operators are binary operators. Note that + and - are used as unary operators too. The operands required for these operations are numeric data. The result of these operations will also be numeric.
Modulus operator (%)
The modulus operator, also called as mod operator, gives the remainder value during arithmetic division. This operator can only be applied over integer operands.
Relational operators
Relational operators are used for comparing numeric data. These are binary operators. The result of any relational operation will be either True or False. In C++, True is represented by 1 and False is represented by 0. There are six relational operators in C++. They are < (less than), > (greater than), == (equal to), <= (less than or equal to), >= (greater than or equal to) and != (not equal to). Note that equality checking requires two equal symbols (==).
Logical operators
Using relational operators, we can compare values. Examples are 3<5, num!=10, etc. These comparison operations are called relational expressions in C++. In some cases, two or more comparisons may need to be combined. In Mathematics we may use expressions like a>b>c. But in C++, it is not possible. We have to separate this into two, as a>b and b>c and these are to be combined using the logical operator &&, i.e. (a>b)&&(b>c). The result of such logical combinations will also be either True or False (i.e. 1 or 0). The logical operators are && (logical AND), || (logical OR) and ! (logical NOT).
Input / Output operators
Usually input operation requires user's intervention. In the process of input operation, the data given through the keyboard is stored in a memory location. C++ provides >> operator for this operation. This operator is known as get from or extraction operator. This symbol is constituted by two greater than symbols. Similarly in output operation, data is transferred from RAM to an output device. Usually the monitor is the standard output device to get the results directly. The operator << is used for output operation and is called put to or insertion operator. It is constituted by two less than symbols.
Assignment operator (=)
When we have to store a value in a memory location, assignment operator (=) is used. This is a binary operator and hence two operands are required. The first operand should be a variable where the value of the second operand is to be stored.
19. Explain different types of expressions in C++.
An expression is composed of operators and operands. The operands may be either constants or variables. All expressions can be evaluated to get a result. This result is known as the value returned by the expression. On the basis of the operators used, expressions are mainly classified into arithmetic expressions, relational expressions and logical expressions.
Arithmetic expressions
An expression in which only arithmetic operators are used is called arithmetic expression. The operands are numeric data and they may be variables or constants. The value returned by these expressions is also numeric. Arithmetic expressions are further classified into integer expressions, floating point (real) expressions and constant expressions.
Integer expressions
If an arithmetic expression contains only integer operands, it is called integer expression and it produces integer result after performing all the operations given in the expression.
Floating point expressions (Real expressions)
An arithmetic expression that is composed of only floating point data is called floating point or real expression and it returns a floating point result after performing all the operations given in the expression.
Relational expressions
When relational operators are used in an expression, it is called relational expression and it produces Boolean type results like True (1) or False (0). In these expressions, the operands are numeric data.
Logical expressions
Logical expressions combine two or more relational expressions with logical operators and produce either True or False as the result. A logical expression may contain constants, variables, logical operators and relational operators.
20. Arrange the fundamental data types in ascending order of size.
Int,Float,Double,Char.
21. The name given to a storage location is known as ___________.
Ans. Memory Address
22. Name a ternary operator in C++.
Ans. Conditional Operator
23. Predict the output of the following operations if x = -5 and y = 3 initially
a. -x
b. -y
c. -x + -y
d. -x - y
e. x % -11
f. x+y
g. x%y
h. x/y
i. x * -y
j. -x %-5
Ans. a. 5
b. -3
c. -(-5)+-3 = 2
d. -(-5)-2 =2
e. -5%-11 = -5
f. -5+3 = -2
g. -5%3 = -2
h. -5/3 = -1
i. -5*-3 =15
j. -(-5)%-5 =0